LightECC is a lightweight elliptic curve cryptography library for its arithmetic for python. It is a hybrid library wrapping many elliptic curve forms such as Weierstrass, Koblitz and Edwards, and many pre-defined curves for those forms. LightECC simplifies these arithmetic operations. You don't need to understand the underlying principles forms. Once you define an elliptic curve in a given form, you can perform addition, subtraction, multiplication, and division of points using standard operators.
The easiest way to install the LightECC package is to install it from python package index (PyPI).
pip install lightecc
Then you will be able to import the library and use its functionalities.
from lightecc import LightECC
Building an elliptic curve cryptosystem is very straightforward in LightECC. You basically need to initialize a LightECC object with a form name and a curve name. By default, it constructs elliptic curves in Weierstras form.After that, you can retrieve the base point of the curve and perform various elliptic curve arithmetic operations, including addition, subtraction, multiplication, and division.
from lightecc import LightECC
# build an elliptic curve
ec = LightECC(
form_name = "edwards", # or weierstrass, koblitz. default is weierstrass.
curve_name = "ed25519", # check out supported curves section
)
# get the base point
G = ec.G
# addition
_2G = G + G
_3G = _2G + G
_5G = _3G + _2G
_10G = _5G + _5G
# subtraction
_9G = _10G - G
# multiplication
_20G = 20 * G
_50G = 50 * G
# division
_25G = _50G / G
Here, addition and subtraction require only basic formulas and can be performed in constant time 𝒪(1).
Supportingly, the double-and-add method is adopted for multiplication, allowing it to be performed very quickly in linear time with 𝒪(log(n)), regardless of the size of the multiplier.
On the other hand, division requires solving the elliptic curve discrete logarithm problem, which is computationally difficult. Its time complexity is 𝒪(n) with brute force.
The order of the elliptic curve is defined by the argument n in the constructed LightECC object. This represents the total number of points on the curve. It also serves as the neutral or identity element in Edwards curves and point at infinity in Weierstass and Koblitz forms, meaning that adding this point to any other point does not change the result. Additionally, elliptic curves exhibit cyclic group properties beyond this point.
ec = LightECC()
# order of elliptic curve
n = ec.n
# neutral element
neutral = n * G
# scalar multiplication
_17G = 17 * G
minus17G = -1 * _17G
# proof of work for neutralism
assert _17G == _17G + neutral
assert _17G + minus17G == neutral
# proof of work for cyclic group
assert (n + 1) * G == G
assert (n + 2) * G == 2 * G
Below is a list of elliptic curves supported by LightECC. Each curve has a specific order (n), which defines the number of points in the finite field. The order directly impacts the cryptosystem's security strength. A higher order typically corresponds to a stronger cryptosystem, making it more resistant to cryptographic attacks.
form | curve | field | n (bits) |
---|---|---|---|
edwards | e521 | prime | 519 |
edwards | id-tc26-gost-3410-2012-512-paramsetc | prime | 510 |
edwards | numsp512t1 | prime | 510 |
edwards | ed448 | prime | 446 |
edwards | curve41417 | prime | 411 |
edwards | numsp384t1 | prime | 382 |
edwards | id-tc26-gost-3410-2012-256-paramseta | prime | 255 |
edwards | ed25519 (default) | prime | 254 |
edwards | mdc201601 | prime | 254 |
edwards | numsp256t1 | prime | 254 |
edwards | jubjub | prime | 252 |
form | curve | field | n (bits) |
---|---|---|---|
weierstrass | bn638 | prime | 638 |
weierstrass | bn606 | prime | 606 |
weierstrass | bn574 | prime | 574 |
weierstrass | bn542 | prime | 542 |
weierstrass | p521 | prime | 521 |
weierstrass | brainpoolp512r1 | prime | 512 |
weierstrass | brainpoolp512t1 | prime | 512 |
weierstrass | fp512bn | prime | 512 |
weierstrass | numsp512d1 | prime | 512 |
weierstrass | eccfrog512ck2 | prime | 512 |
weierstrass | gost512 | prime | 511 |
weierstrass | bn510 | prime | 510 |
weierstrass | bn478 | prime | 478 |
weierstrass | bn446 | prime | 446 |
weierstrass | bls12-638 | prime | 427 |
weierstrass | bn414 | prime | 414 |
weierstrass | brainpoolp384r1 | prime | 384 |
weierstrass | brainpoolp384t1 | prime | 384 |
weierstrass | fp384bn | prime | 384 |
weierstrass | numsp384d1 | prime | 384 |
weierstrass | p384 | prime | 384 |
weierstrass | bls24-477 | prime | 383 |
weierstrass | bn382 | prime | 382 |
weierstrass | curve67254 | prime | 380 |
weierstrass | bn350 | prime | 350 |
weierstrass | brainpoolp320r1 | prime | 320 |
weierstrass | brainpoolp320t1 | prime | 320 |
weierstrass | bn318 | prime | 318 |
weierstrass | bls12-455 | prime | 305 |
weierstrass | bls12-446 | prime | 299 |
weierstrass | bn286 | prime | 286 |
weierstrass | brainpoolp256r1 | prime | 256 |
weierstrass | brainpoolp256t1 | prime | 256 |
weierstrass | fp256bn | prime | 256 |
weierstrass | gost256 | prime | 256 |
weierstrass | numsp256d1 | prime | 256 |
weierstrass | p256 | prime | 256 |
weierstrass | secp256k1 (default) | prime | 256 |
weierstrass | tom256 | prime | 256 |
weierstrass | bls12-381 | prime | 255 |
weierstrass | pallas | prime | 255 |
weierstrass | tweedledee | prime | 255 |
weierstrass | tweedledum | prime | 255 |
weierstrass | vesta | prime | 255 |
weierstrass | bn254 | prime | 254 |
weierstrass | fp254bna | prime | 254 |
weierstrass | fp254bnb | prime | 254 |
weierstrass | bls12-377 | prime | 253 |
weierstrass | curve1174 | prime | 249 |
weierstrass | mnt4 | prime | 240 |
weierstrass | mnt5-1 | prime | 240 |
weierstrass | mnt5-2 | prime | 240 |
weierstrass | mnt5-3 | prime | 240 |
weierstrass | prime239v1 | prime | 239 |
weierstrass | prime239v2 | prime | 239 |
weierstrass | prime239v3 | prime | 239 |
weierstrass | secp224k1 | prime | 225 |
weierstrass | brainpoolp224r1 | prime | 224 |
weierstrass | brainpoolp224t1 | prime | 224 |
weierstrass | curve4417 | prime | 224 |
weierstrass | fp224bn | prime | 224 |
weierstrass | p224 | prime | 224 |
weierstrass | bn222 | prime | 222 |
weierstrass | curve22103 | prime | 218 |
weierstrass | brainpoolp192r1 | prime | 192 |
weierstrass | brainpoolp192t1 | prime | 192 |
weierstrass | p192 | prime | 192 |
weierstrass | prime192v2 | prime | 192 |
weierstrass | prime192v3 | prime | 192 |
weierstrass | secp192k1 | prime | 192 |
weierstrass | bn190 | prime | 190 |
weierstrass | secp160k1 | prime | 161 |
weierstrass | secp160r1 | prime | 161 |
weierstrass | secp160r2 | prime | 161 |
weierstrass | brainpoolp160r1 | prime | 160 |
weierstrass | brainpoolp160t1 | prime | 160 |
weierstrass | mnt3-1 | prime | 160 |
weierstrass | mnt3-2 | prime | 160 |
weierstrass | mnt3-3 | prime | 160 |
weierstrass | mnt2-1 | prime | 159 |
weierstrass | mnt2-2 | prime | 159 |
weierstrass | bn158 | prime | 158 |
weierstrass | mnt1 | prime | 156 |
weierstrass | secp128r1 | prime | 128 |
weierstrass | secp128r2 | prime | 126 |
weierstrass | secp112r1 | prime | 112 |
weierstrass | secp112r2 | prime | 110 |
form | curve | field | n (bits) |
---|---|---|---|
koblitz | b571 | binary | 570 |
koblitz | k571 | binary | 570 |
koblitz | c2tnb431r1 | binary | 418 |
koblitz | b409 | binary | 409 |
koblitz | k409 | binary | 407 |
koblitz | c2pnb368w1 | binary | 353 |
koblitz | c2tnb359v1 | binary | 353 |
koblitz | c2pnb304w1 | binary | 289 |
koblitz | b283 | binary | 282 |
koblitz | k283 | binary | 281 |
koblitz | c2pnb272w1 | binary | 257 |
koblitz | ansit239k1 | binary | 238 |
koblitz | c2tnb239v1 | binary | 238 |
koblitz | c2tnb239v2 | binary | 237 |
koblitz | c2tnb239v3 | binary | 236 |
koblitz | b233 | binary | 233 |
koblitz | k233 | binary | 232 |
koblitz | ansit193r1 | binary | 193 |
koblitz | ansit193r2 | binary | 193 |
koblitz | c2pnb208w1 | binary | 193 |
koblitz | c2tnb191v1 | binary | 191 |
koblitz | c2tnb191v2 | binary | 190 |
koblitz | c2tnb191v3 | binary | 189 |
koblitz | b163 | binary | 163 |
koblitz | c2pnb163v1 | binary | 163 |
koblitz | k163 (default) | binary | 163 |
koblitz | ansit163r1 | binary | 162 |
koblitz | c2pnb163v2 | binary | 162 |
koblitz | c2pnb163v3 | binary | 162 |
koblitz | c2pnb176w1 | binary | 161 |
koblitz | sect131r1 | binary | 131 |
koblitz | sect131r2 | binary | 131 |
koblitz | sect113r1 | binary | 113 |
koblitz | sect113r2 | binary | 113 |
koblitz | wap-wsg-idm-ecid-wtls1 | binary | 112 |
Explore the LightPHE
library for its encryption and decryption implementation, which also demonstrates additive homomorphic properties with Elliptic Curve ElGamal algorithm. Additionally, check out LightDSA
for elliptic curve based digital signature implementations such as ECDSA and EdDSA. These are both built on LightECC's fundamentals.
All PRs are more than welcome! If you are planning to contribute a large patch, please create an issue first to get any upfront questions or design decisions out of the way first.
You should be able run make test
and make lint
commands successfully before committing. Once a PR is created, GitHub test workflow will be run automatically and unit test results will be available in GitHub actions before approval.
There are many ways to support a project - starring⭐️ the GitHub repo is just one 🙏
You can also support this work on Patreon, GitHub Sponsors or Buy Me a Coffee.
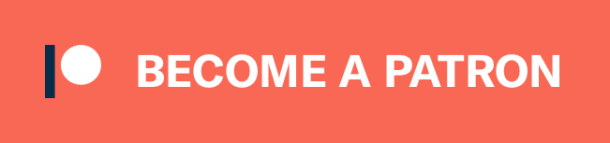
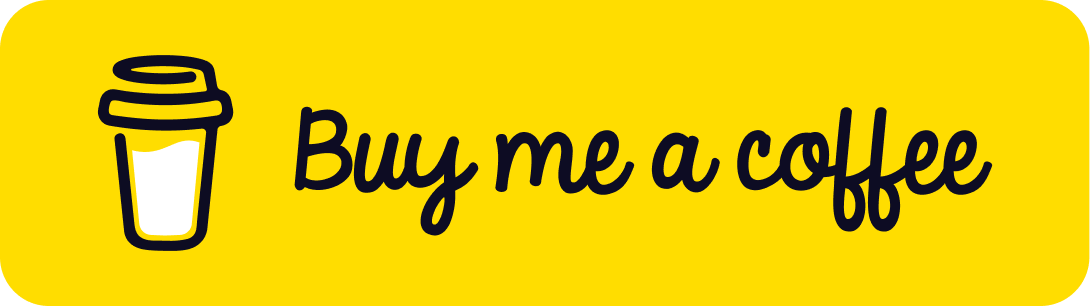
Also, your company's logo will be shown on README on GitHub if you become sponsor in gold, silver or bronze tiers.
Please cite LightECC in your publications if it helps your research. Here is its BibTex entry:
@misc{serengil2025lightecc
author = {Serengil, Sefik Ilkin},
title = {LightECC: A Lightweight Elliptic Curve Cryptography Arithmetic Library for Python with Support for Prime and Binary Fields},
year = {2025},
publisher = {GitHub},
howpublished = {https://github.com/serengil/LightECC},
}
LightECC is licensed under the MIT License - see LICENSE
for more details.
LightECC's logo, designed by Identidea, is inspired from Edwards curves graphs.